Connected account registrations
Refer merchants to Helcim for processing using your unique affiliate URL.
Using our connected account registration process and webhook events, we can link merchants who sign up through your partner registration URL with your systems unique identifier for that merchant. This way we can communicate the outcome of that referral registration and send the merchants api-token
to store securely in your system for processing if the merchant account is approved.
In addition to connected account registrations and webhooks, you can also make use of additional optional functionality for pre-filling your connected account registration page and co-branding it so your customers recognize the page as being associated with your business.
Connected account registrations
After registering to become a partner through our partner portal, the Helcim Partnership team will provide you with your partner-token
.
When you refer merchants to Helcim, you will send them to a unique URL for their registration. This registration URL contains two additional parameters for a partner token and a connected account identifier, and should look something like this.
// Example format for the partner registration URL
'https://hub.helcim.com/signup/register?pt="partner_token"&cid="connected_account_identifier"'
- The "pt" parameter value for "partner_token", should be the
partner-token
provided to you by our Partnerships team, and will be the same for every registration. - The "cid" parameter value for "connected_account_identifier", should be the unique value you use to identify the referred merchant in your system, and will change for every unique registration.
When we receive a registration in our system through your registration link using these two URL parameters, the referred merchant becomes a "Connected Account" and the webhook notification process is triggered.
Through this webhook process we will notify you of the outcome of the merchants application, as well as their merchant api-token
if approved, so that you can process on that merchants behalf through your integration.
Testing connected account registrations
The connected account registration process does not currently have a sandbox in which you can register test accounts, so registrations completed through your partner registration URL will create production Helcim accounts.
We recommend when testing the connected account registration process that you include the word "TEST" at the start of the legal and operating business names, then connect with your implementation and partnership specialists with the CID for the given test account so that they can review and approve the account.
Connected Account Webhooks
Review our documentation for Connected Account Webhook Events, so that you are automatically notified when a referred merchant is approved and receive their
api-token
in a secure manner.
Pre-filled registrations
If you're collecting business information from the merchants you refer to Helcim when they sign up for your platform, then you can pre-fill the partner registration form and send that data to Helcim using a single API call.
Pre-filling the registration form reduces the amount of effort required for a potential connected account to sign up for Helcim's services, by presenting users with a signup application that is pre-filled with information previously provided to partners.
Information in the form is not locked and the person registering for the account can remove or amend the values of any pre-filled field, so long as the validation and submission requirements of the registration form are met.
Request endpoint
POST requests with connected account details should be sent to https://api.helcim.com/v2/applications/prefill.
Request authorization
All requests must contain the partner api-token
in the header of the request. Your partner api-token
will be provided through a secure 1Password link emailed to a person of your choosing.
The partner
api-token
is different from yourpartner-token
or the merchantapi-token
, and is only needed for requests to Partner API endpoints.If you have not received your partner
api-token
yet, then please reach out to your Partnership Specialist for support.
The connectedAccountId
should be generated by the partner, however they would like to keep track of their connected accounts. At this point in time, Helcim accepts a connectedAccountId
made up of letters, numbers, underscores, and hyphens, to a 255 character limit.
Request fields
We currently support the pre-filling of the following fields of signup.
Parameter | Type | Requirement | Description |
---|---|---|---|
| string | mandatory | The partners chosen connected account ID that references the referred merchant within their system. Can contain letters, numbers, hyphens, and underscores, up to 255 characters. |
| string | mandatory | An RFC 5322 valid email address for the primary person who will complete the pre-filled registration for the merchant. |
| string | optional | The known business operating name for the merchant. |
| string | optional | The legal registered business name for the merchant. |
| integer | optional | The year of registration for the merchant, in YYYY format. |
| string | optional | Choose from the following options:
|
| string | optional | 10 digits, without country code (+1), but brackets, dots, spaces allowed. Examples include:
|
| string | optional | Processing currency of "CAD" or "USD". US Based business may only process in USD, where Canadian based business may process in either CAD or USD. |
string | optional | Business website or social media link. | |
| object | optional | Contains information for the businesses legal registered address. |
| string | optional | Valid non-empty string for the primary address values for the business, such as "123 Example Street NW". |
| string | optional | Valid non-empty string for the secondary address values for the business, such as "Suite 2". |
| string | optional | Valid non-empty string for the city that the business operates in. |
| string | optional | Valid two letter State or Province code for the business, such as "NY" "AB", or "CA". |
| string | optional | Country the business operates in. Either "Canada" or "USA" are accepted for referred merchants. |
| string | optional | Valid zip or postal code of the address for the business, containing 5 digits in a row (US) or 6 alternating letter/number (Canada) that is not case sensitive and may contain a space between first 3 characters and last 3 characters. |
Request example
// Example pre-filled registration POST request
const options = {
method: 'POST',
headers: {
accept: 'application/json',
'content-type': 'application/json',
Authorization: Bearer {your_partner_api_token}
},
body: JSON.stringify({
"connectedAccountId": "partnerIdValue1000",
"emailAddress": "[email protected]",
"businessOperatingName": "Example Corp",
"businessLegalName": "Example Corporation",
"businessYearRegistered": 1989,
"businessType": "INCORPORATED",
"businessPhoneNumber": "(123)-123-1234",
"businessWebsite": "www.example.com",
"processingCurrency": "USD",
"businessAddress": {
"street1": "1st Street",
"street2": "Apt #1",
"city": "New York",
"province": "NY",
"country": "USA",
"postalCode": "10009"
}
})
};
fetch('https://api.helcim.com/v2/applications/prefill', options)
.then(res => res.json())
.then(res => console.log(res))
.catch(err => console.error(err));
Successful API response
The Pre-filled Registration endpoint will respond to your requests with one of the following:
For valid requests that have been successfully processed, the HTTP response code will be 201 Created
and the response payload will be the following:
{
"message": "Successfully saved registration information"
}
After a you receive a successful response to your request to pre-fill connected account information, you can then direct potential connected accounts to sign up through your partner registration URL.
// Example format for the partner registration URL
'https://hub.helcim.com/signup/register?pt="partner_token"&cid="connected_account_identifier"'
Errors and error handling
If there are validation issues, the response will contain information on which fields were invalid and the acceptable formats for those fields. For example, for a request payload that contains an invalid email address and business registration year, the HTTP response code will be 400 Bad Request
and the response payload will be the following:
{
"timestamp": "2024-11-01T16:24:31+00:00",
"status": "error",
"errors": {
"ERR_INVALID_REQUEST": [
{
"code": "ERR_INVALID_REQUEST",
"source": "emailAddress",
"data": "[][email protected]",
"message": "emailAddress is a required field and must be in the format: [email protected]"
}
]
}
}
In the case of failed authentication, the HTTP response code will be 401 Unauthorized and the response payload will be the following:
{
"timestamp": "2024-11-01T16:24:31+00:00",
"status": "error",
"errors": {
"ERR_UNAUTHORIZED": [
{
"code": "ERR_UNAUTHORIZED",
"message": "You are not authorized to access this resource."
}
]
}
}
For any server issues that result in the request not being processed successfully, the HTTP response code will be 500 Internal Server Error and the response payload will be the following:
{
"timestamp": "2024-11-01T16:24:31+00:00",
"status": "error",
"errors": {
"ERR_INTERNAL": [
{
"code": "ERR_INTERNAL",
"message": "An internal error has occurred"
}
]
}
}
Co-branded registrations
Through Helcim's co-branded registrations, you can reduce the friction for referred merchants signing up for their Helcim account, by keeping a consistent brand presence through their registration for services with Helcim.
In order to have your partner registration URL co-branded, please provide your Helcim Partnership Specialist with the following.
- Logo and brand guideline documentation, including the HEX code for your primary brand colour.
Once we receive these assets, Helcim will configure your partner registration URL to match your branding in addition to ours. Once your co-branded page is ready to go, the Partnerships Team will notify you.
In order for co-branded registrations to be displayed, please ensure that your partner-token
URL parameter in your partner registration URL is correct for all merchants referred to Helcim for processing. This is what our system is looking for to present the co-branded version of the registration.
https://hub.helcim.com/signup/register?pt=<partner_token>&cid=<abc123def456>
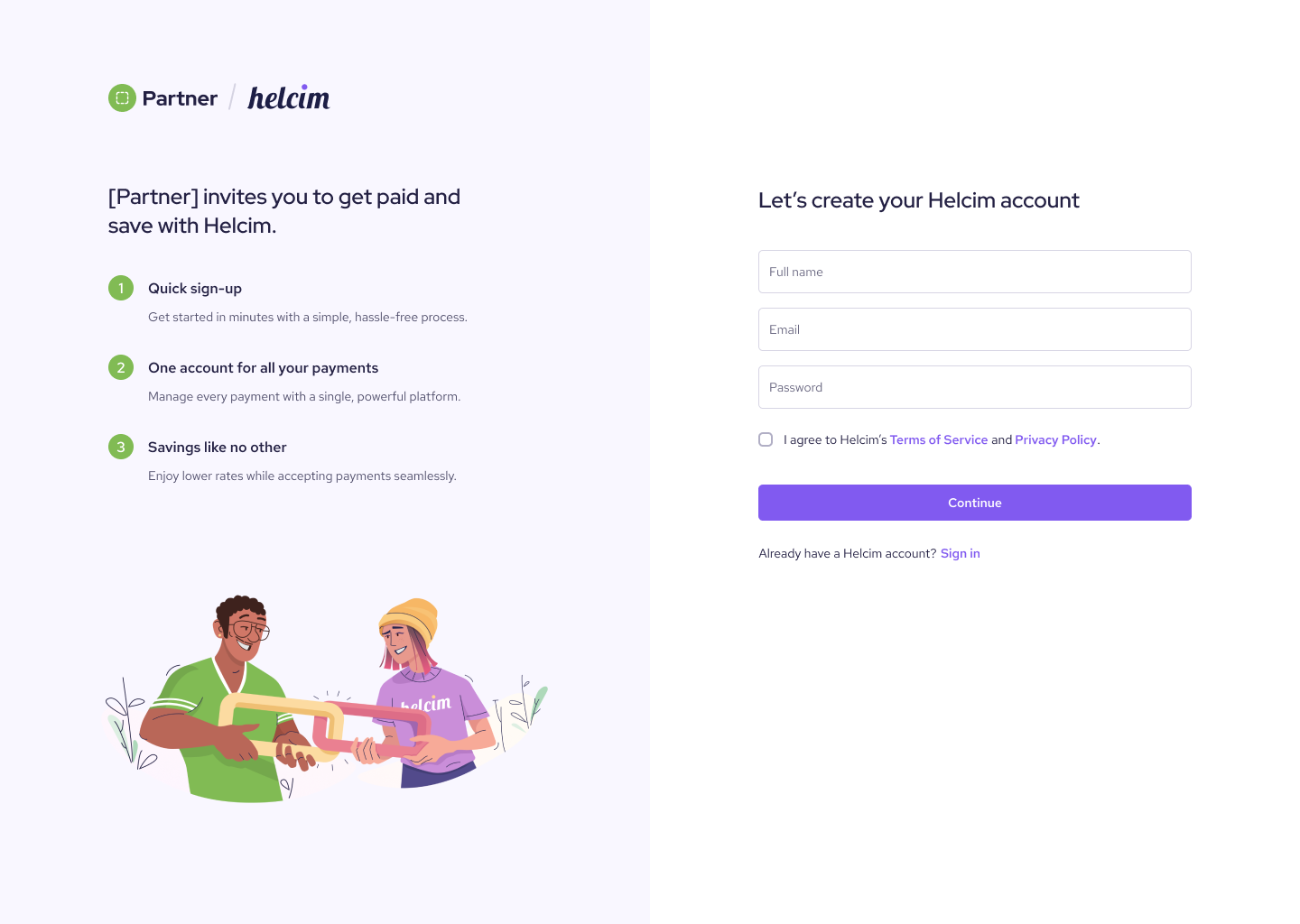
Updated about 2 months ago