Customers
Track customer information and payments in the Helcim system.
The customer object
A customer
object represents a unique customer in the Helcim system, which could be either an individual or a business.
The customer stores a number of the core attributes that apply to customers, including:
- A unique
customerCode
value to be used as a reference for your customer in the Helcim system. - A required
contactName
and / orbusinessName
, as well as an optionalcellPhone
contact number for your customer. - A
billingAddress
andshippingAddress
sub-object to store your customers billing and shipping details.
// Example customer object
{
"id": 24332090,
"customerCode": "CST1049",
"businessName": "Helcim",
"contactName": "John Doe",
"dateCreated": "2024-09-18 15:26:25",
"dateUpdated": "2024-09-18 15:26:26",
"cellphone": "1234567899",
"billingAddress": {
"name": "John Doe",
"street1": "123 Test Street",
"street2": "Suite 2",
"city": "Calgary",
"province": "AB",
"country": "CAN",
"postalCode": "H0H0H0",
"phone": "1234567899",
"email": "[email protected]"
},
"shippingAddress": {
"name": "John Doe",
"street1": "123 Test Street",
"street2": "Suite 2",
"city": "Calgary",
"province": "AB",
"country": "CAN",
"postalCode": "H0H0H0",
"phone": "1234567899",
"email": "[email protected]"
},
"cards": []
}
Retrieving objects in the cards array
To retrieve
card
objects associated with a customer in the Helcim system, you can use the following endpoints.
Creating a customer
There are a few different ways to create a customer in the Helcim system, including:
- Using the Create customer endpoint in the Customer API.
- Using the
customerRequest
object in your HelcimPay.js initialize request. - Using the default Helcim system functionality that will create a basic
customer
object for any payments processed where an existing customer is not linked to the transaction.
Either a contactName
or businessName
parameter (or both) are the minimum required body parameters when creating a customer
object.
The optional body parameters can include the following values.
Key | Type | Description |
---|---|---|
customerCode | string | A string value used as a reference for the customer in the Helcim system. If not passed, the Helcim system will automatically generate this value based on your Customer Settings. |
cellPhone | string | A string value between 10 and 16 characters long, used to store the customer contact phone number. |
billingAddress | object | A sub-object used to store the customers billing information. A valid billingAddress object must contain name , street1 , and postalCode string values. |
shippingAddress | object | A sub-object used to store the customers shipping information. A valid shippingAddress object must contain name , street1 , and postalCode string values. |
Setting the
billingAddress
andshippingAddress
province and country values
- The
province
andcountry
values for acustomer
object are used for tax calculation purposes, when tax is calculated using customer location in the Helcim system.- It is optional to send these values, but if you send either a
province
orcountry
value for the United States or Canada, the other value becomes required and must also be sent.
Associated objects
A customer
object created in the Helcim system can be linked to invoice
, transaction
, and subscription
objects, allowing for a history of customer payments and records.
You can link an existing customer to the following types of objects.
- Transactions created through the Payment API or HelcimPay.js using the
customerCode
. - Transactions created through the ACH Payment API using the
customerId
. - Invoices created through the Invoice API using the
customerId
. - Subscriptions created through the Recurring API using the
customerCode
.
Customer settings
Your Helcim account Customer Settings will impact the customerCode
created for any default customer object created by payments through the Payment API or HelcimPay.js.
These settings, located under All Tools and then Settings, control the default Prefix and Number Length of your customerCode
values.
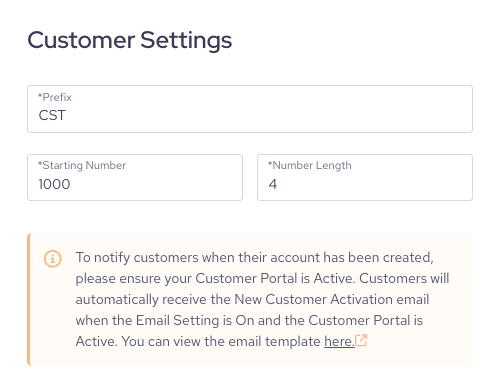
Customer endpoints
Create, retrieve, and update customer
objects using the following endpoints.
Endpoint | Description |
---|---|
Create customer | Create a new customer to tracking customer information and payments. |
Get customer | Retrieve a single customer based on the customerId |
Get customers | Retrieve an array of customer objects based on a range of query parameters. |
Update customer | Update a single customer based on the customerId . |
Updated about 2 months ago