Bank accounts
Store customer bank accounts for quick and easy processing.
The bank account object
A bank
object is created and stored against a customer
object, when any payment is processed through the Helcim system with ACH bank details. A customer's bank information can be saved through a range of Helcim payment tools, including HelcimPay.js, Payment Pages, Virtual Terminal, and more.
A bank account stores a number of core payment details for the customer, including:
- Non-sensitive bank holder details, such as account type, institution and transit numbers for Canadian bank details, or routing for US bank details, as well as the last 4 digits of the bank account number.
- A unique
bankToken
value to allow processing within the ACH Payment API. - A range of date values indicating when the bank account was created, last updated, last used, and verified.
- Whether the bank account details are verified and ready to process payments.
- Address details for the bank account details.
// Example bank account object
{
"id": 53539,
"customerId": 22745923,
"dateCreated": "2024-07-05 09:50:13",
"dateUpdated": "2024-07-05 09:50:13",
"dateLastUsed": "0000-00-00 00:00:00",
"dateVerified": "2024-07-05 09:50:13",
"bankToken": "d6ad2df48c348351152bbd",
"accountType": "CHECKING",
"accountCorporate": "PERSONAL",
"verified": 1,
"ready": 1,
"bankIdNumber": "003",
"transitNumber": "23456",
"routingNumber": "",
"bankAccountNumberL4": "6789",
"address": {
"name": "John Doe",
"street1": "123 Test Street",
"street2": "",
"city": "Calgary",
"province": "AB",
"country": "CAN",
"postalCode": "H0H0H0",
"phone": "",
"email": ""
}
}
Capturing customer bank account details
The Helcim system will verify and tokenize any bank account information used to process a payment through our payment tools, storing this against a customer for later processing.
- If a payment is linked to an existing customer before processing, the payment and bank account details used for that payment will also be stored against the
customer
object. - If a payment is not linked to an existing customer before processing, the Helcim system will create a basic
customer
object to store the payment details against.
Using the ACH Payment API or HelcimPay.js to Capture Bank Account Details
Capturing customer bank account details can be completed using the ACH Payment API or HelcimPay.js, to create a unique
bankToken
value that can be used to process payments within the Helcim system.
The Customer API contains endpoints that allow you to create, retrieve and modify Bank account objects, request banking information from your customers, or retrieve and modify PAD agreements. To test requests to these endpoints you can use our API Reference section for the Customer API here.
How to create a customer bank account
You can create a new Bank account object in the Helcim system using the Create a bank account endpoint. A bankAccount
object is associated with a single customer
object, but can have one of more related PAD agreements of varying states.
- Canadian merchants will need to provide the
bankAccountNumber
,bankFinancialNumber
, andbankTransitNumber
for a valid Canadian bank account used by the customer. - US merchants will need to provide the
bankAccountNumber
andbankRouting
number for a valid United States bank account used by the customer. - The values passed in this request need to match the customers bank records exactly. Discrepancies in these values may result in the customers bank rejecting any attempted ACH transactions.
Before using this endpoint
You will need to have an existing customer
object that you would like to associate the bank account with, by passing the relevant customerId
in the path parameter of your request. The Customer API can be used to create, retrieve, or modify a customer
object to be used in this request.
We require a valid
billingAddress
object for the customer.This email address is used by the Helcim system to send a bank authorization email to the customer on successful bank account creation.
Their approval of this authorizes transactions using these banking details and creates a valid approved pre-authorized debit agreement for the banking information.
Failure to obtain an approved authorization may result in your ACH transactions being declined by the customers bank.
Understanding create a customer bank account responses
When a successful request is made to create a bankAccount
object, the Helcim system will respond with a JSON object containing the id
value for the object and a success message
indicating the object was created and an authorization email was sent.
Full bankAccount
details can be retrieved by sending a request to the Get customer bank account endpoint with the customerId
and bank account id
returned in this response.
{
"bankAccount": {
"id": 45367
},
"message": "Successfully created new bank account with ID #45367. A bank authorization email was also emailed to [email protected]."
}
Attempting to create a bankAccount
object for a customer that does not have a valid email address on file will result in an error response. An email should be added using the Update customer endpoint.
{
"message": "Customer email address on file () is invalid."
}
How to request bank account information from a customer
As an alternative to the Create a bank account endpoint, the Request bank account information from a customer endpoint can be used to send a bank authorization email from the Helcim system to the billing email listed in the customer
object.
The endpoint will respond with a success message
, or an error if no email address is included in the customer
object.
{
"message": "A bank authorization email was emailed to [email protected]."
}
Adding new banking information
Where a customer
object does not have existing bank account information, the authorization email will prompt the customer enter their banking information and approve a PAD agreement.
Saving the banking information will create a new bankAccount
object and PAD agreement for the customer in question.
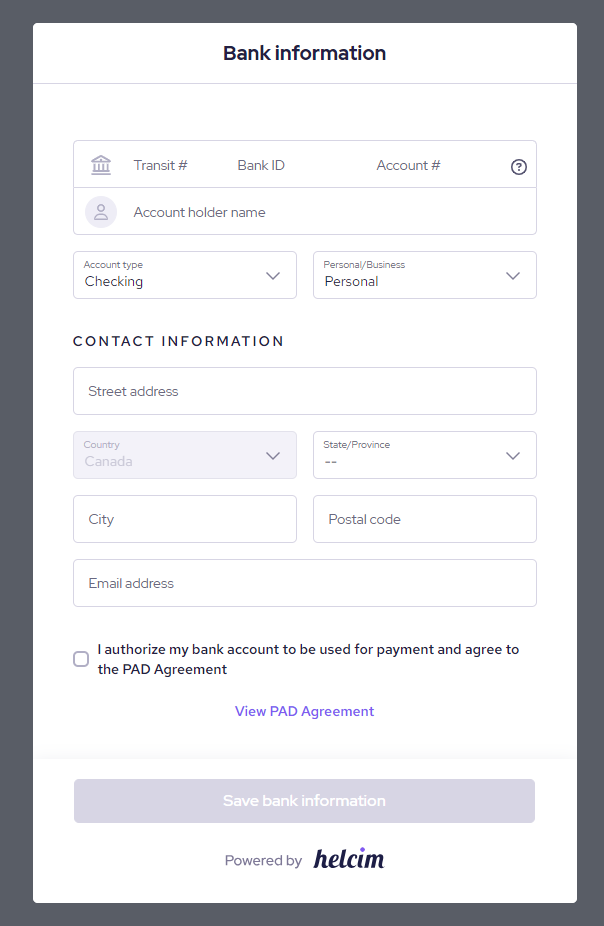
Because bankAccount
objects created through this action are completed by the customer, you will not have a bank account id
value returned to you. We recommend using the Get customer bank accounts endpoint to retrieve their banking information once you receive the email confirmation that the bank authorization was approved.
Adding additional banking information
Allow your customers to update or add additional banking information to a customer
object, in the event their banking details have changed. Through the authorization email, the customer can select the dropdown and then click the Add new bank option to enter and authorize new banking details.
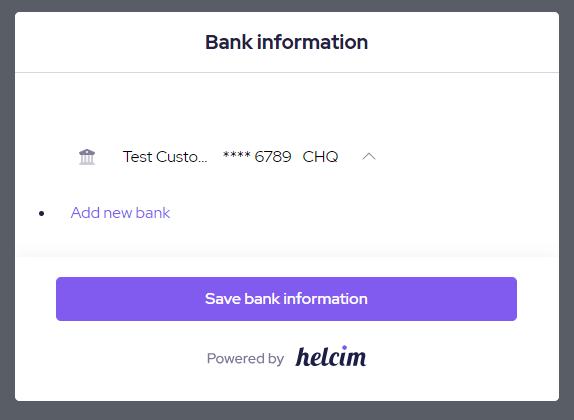
Adding new banking information through this method will not set the new bankAccount
object as the default for processing. This can be completed by calling the Set customer bank account as default endpoint and passing the relevant customerId
and bankAccountId
values.
Verifying existing banking information
This endpoint can be used to verify existing banking information in the following circumstances.
- The customer in question has an existing
bankAccount
object on file with either no PAD agreement, a PAD agreement that has not been approved, or a PAD agreement that has been revoked. - The customer did not receive the original bank authorization email triggered by the Create a bank account endpoint.
Verifying existing banking information through this process will create a new PAD agreement and authorize their banking information for processing payments.
Understanding verified and ready statuses
A bank
object in the Helcim system has two parameters that dictate whether the bank account information is valid and ready to process payments.
Parameter | Description |
---|---|
verified | Banking that is verified has been validated and signed off by the Helcim team. ACH payments will still go through a second level of verification by the customers bank during the settlement process. |
ready | Banking that is ready has been approved by the customer through an authorized PAD agreement. |
How to retrieve customer bank accounts
The Customer API contains two endpoints that will allow you to retrieve bankAccount
objects for a relevant customer in the Helcim system.
- Where the
customerId
andbankAccountId
are known, you can call the Get customer bank account endpoint to retrieve the details of a specificbankAccount
object associated to that customer. - Where a customer may have multiple bank accounts or the
bankAccountId
is not known, you can call the Get customer bank accounts endpoint to retrieve an array ofbankAccount
objects associated to that customer.
These endpoints will return the bankAccount
object, or an array of objects, with the information outlined in the example below.
{
"id": 41992,
"customerId": 19411033,
"dateCreated": "2024-03-14 16:51:38",
"dateUpdated": "0000-00-00 00:00:00",
"dateLastUsed": "0000-00-00 00:00:00",
"dateVerified": "0000-00-00 00:00:00",
"bankToken": "3f747d045aab557481cd1f",
"accountType": "CHECKING",
"accountCorporate": "PERSONAL",
"verified": 0,
"ready": 0,
"bankIdNumber": "123",
"transitNumber": "12345",
"routingNumber": "",
"bankAccountNumberL4": "6789",
"address": {
"name": "Test Customer",
"street1": "123 Test Street",
"street2": "",
"city": "Calgary",
"province": "AB",
"country": "CAN",
"postalCode": "H0H 0H0",
"phone": "",
"email": ""
}
}
How to set a customer bank account as the default
Creating a new bankAccount
object in the Helcim system does not automatically set that object as the default for processing. A default bank account is the account that would be shown as a priority, or processed on automatically, through other Helcim payment tools such as Pay Now Invoices or Subscriptions.
If you allow a customer to indicate they would like a bank account to be set as default in your system, then you can call the Set customer bank account as default endpoint to complete that corresponding action in the Helcim system.
Bank account endpoints
Create, request, and retrieve bank account information, and set a new default bank account for a customer, using the following endpoints.
Endpoint | Description |
---|---|
Create a bank account | Create a new bank object for a customer to process ACH bank payments. |
Request bank account information from a customer | Request bank account information from a customer to create a new bank object for processing ACH bank payments. |
Get customer bank account | Retrieve a customer bank object, based on customerId and bankAccountId . |
Get customer bank accounts | Retrieve an array of customer bank objects, based on customerId . |
Set customer bank account as default | Set a customer bank account as the default account for processing. Default accounts will be used for all recurring payments and display as the primary account in many Helcim payment tools. |
Updated 19 days ago