Initialize a HelcimPay.js Checkout Session
Before you can render the HelcimPay.js payment modal, you must first perform an API call from your website or applications back-end server to initialize your HelcimPay.js checkout session and get your checkoutToken
and secretToken
.
Creating your API Access Configuration
All implementations of HelcimPay.js will require an API Access Configuration.
This generates your unique
api-token
that is used to authenticate your Initialization requests with the Helcim API and sets the permissions for that token to process through HelcimPay.js.
HelcimPay.js Initialize Request
The HelcimPay.js initialize request is how you will create your HelcimPay.js checkout session and configure the unique features and functionality for your customers payment experience.
The initialize request creates a checkoutToken
that controls a number of parameters that are rendered in the payment modal, including:
- What payment type and payment method the customer is allowed to process payment with, including the amount and currency of the payment.
- Whether the checkout session is linked to an existing customer or invoice, or whether you will use the
customerRequest
andinvoiceRequest
objects to create new ones on successful payment. - Whether you would like to use Helcim Fee Saver to pass on your credit card processing fees, or let your customer pay with ACH payment to reduce your processing costs.
- Whether your customer is allowed to make partial payments for their invoice amount.
- Whether you want to hide existing payment details stored in your Helcim card vault for that customer, or whether you want to set new payment details being used as the customers default moving forward.
Important Note
When initializing HelcimPay.js, this request should be made from your website or applications secure back-end server. Attempting to send your HelcimPay.js initialize request from your front-end or client side code, will result in a Cross-Origin Resource Sharing (CORS) error.
Configuring your HelcimPay.js Payment
Through your HelcimPay.js initialize request, you can control a range of variables related to the payment you would like your customer to process through their checkout session.
Using the paymentMethod
parameter you can determine if your customer is shown only the credit card or ACH bank payment fields based on your preferences, or if they are shown both so that they can choose their preferred payment method. The paymentMethod
that you select determines the paymentType
that is available for your customer.
- When initialized with a
paymentMethod
of "cc" or "cc-ach", you have access to the purchase, preauth, and verify payment types. - When initialized with a
paymentMethod
of "ach", you have access to purchase or verify payment types. - When initialized without a
paymentMethod
the checkout session will default to "cc" only.
Payment Type | Description |
---|---|
purchase | Will process an immediate one-time payment for the amount specified in your initialize request, against the credit card or bank details provided by the customer. |
preauth | Will process a pre authorized payment for the amount specified in your initialize request against the credit card provided by the customer. Can be captured using the Process Capture Transaction endpoint in the Payment API, or manually in the Payments section of your Helcim account. |
verify | Will process a verify payment for an amount of 0, that verifies and tokenizes the credit card or bank details provided by the customer. Returns a cardToken or bankToken value in the payment response that can be used to process future payments securely through the Payment API. |
The amount
value passed in your initialize request will determine the amount displayed in the payment modal to the customer. A verify payment must have an amount
value of 0. If initializing with Helcim Fee Saver, the convenience fee amount is additional to your base amount.
The currency
value passed in your initialize request will determine the currency of the payment processed.
- Canadian based merchants may process credit card payments with either "CAD" by default, or "USD" if their Helcim accounts are configured to allow multi currency processing. ACH payments will only process in "CAD", the core local currency of your Helcim business account, regardless of the
currency
value passed. - US based merchants may process credit card and ACH payments in "USD" only.
The taxAmount
value passed in your initialize request can be used to determine whether level 2 processing rates are applied to eligible transactions. This parameter should be included in the checkout session amount
value and does not automatically increase that value is passed.
Cross Border ACH Payments
The Helcim system does not allow cross border ACH payments. All ACH payments for Canadian or US based merchants must be processed in the core local currency of their Helcim business account.
Creating a Customer with HelcimPay.js
The HelcimPay.js initialize request allows you to pass a customerRequest
object that will be used by the Helcim system to:
- Create a
customer
object on approved or declined payment that the payment is linked to, using the parameters passed in the request. - Prepopulate the Cardholder name and AVS Street address and ZIP / Postal code fields in the payment modal.
The customerRequest
object allows you to set the following values:
- A unique
customerCode
reference for the customer, that may match your system - A
contactName
that is saved in thecustomer
object and used to prepopulate the Cardholder name field in the payment modal, as well as an optionalbusinessName
andcellPhone
. - An optional
billingAddress
sub-object that is saved in thecustomer
object and used to prepopulate the Street address and ZIP / Postal code fields in the payment modal - An optional
shippingAddress
sub-object that that is saved in thecustomer
object.
If a customerRequest
object is not passed in the initialize request, the Helcim system will create a basic customer
object based on your default customer settings and the payment details passed by the customer in the modal.
Creating an Invoice with HelcimPay.js
The HelcimPay.js initialize request allows you to pass a invoiceRequest
object that will be used by the Helcim system to:
- Create an
invoice
object on successful payment that the payment is linked to, using the parameters passed in the request.
The invoiceRequest
object allows you to set the following values:
- A unique
invoiceNumber
reference for the invoice, that may match your system. - Whether the invoice created has a
tipAmount
ordepositAmount
. - Whether the invoice has any
shipping
,pickup
,tax
, ordiscounts
objects. - An array of objects containing any
lineItems
objects.
The total amount due on an invoice
object created by an invoiceRequest
in your HelcimPay.js initialization, can be for the same amount or a different amount the the payment amount
passed. If the sum of the different amounts in the invoiceRequest
is greater than your payment amount
, the invoice will remain with a status
of due until the full amount is paid.
If a invoiceRequest
object is not passed in the initialize request, the Helcim system will create a basic object based on your default invoice settings.
Linking to an Existing Customer or Invoice
If you have an existing customer or invoice in the Helcim system that you would like to link the payment to, you can utilize the customerCode
and invoiceNumber
parameters. If you pass both a customerCode
and invoiceNumber
, the invoice must be associated and linked to the customer object first. You can use the Update invoice endpoint.
Passing an existing customerCode
with stored payment details will result in the payment modal pre-populating those details, which is a great way to make subsequent checkouts efficient for your customers. To link a payment to a customer but hide any stored payment details, review the documentation to Hide Existing Payment Details.
Allowing Partial Payment of Invoices
The allowPartial
parameter can be used to let your customers make partial payments on existing invoices linked to your HelcimPay.js checkout session via their invoiceNumber
. In order to allow partial payments on invoices you must:
- Ensure that the invoice that is linked has a
status
of "DUE" - Ensure that the "Allow Partial Payment" setting is enabled in your Helcim account Settings. This is located under All Tools, Settings, Invoice Settings.
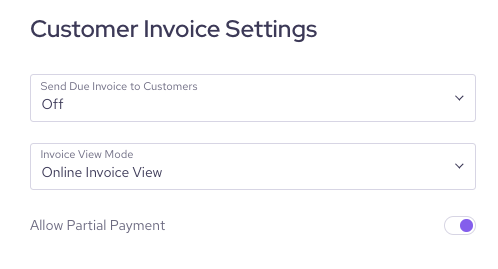
From inside the payment modal the customer can then select to pay the invoice in full, or toggle on Partial Payment. With partial payment toggled on the modal will allow the customer to pay either a partial amount, or a percentage, that is due for the invoice.
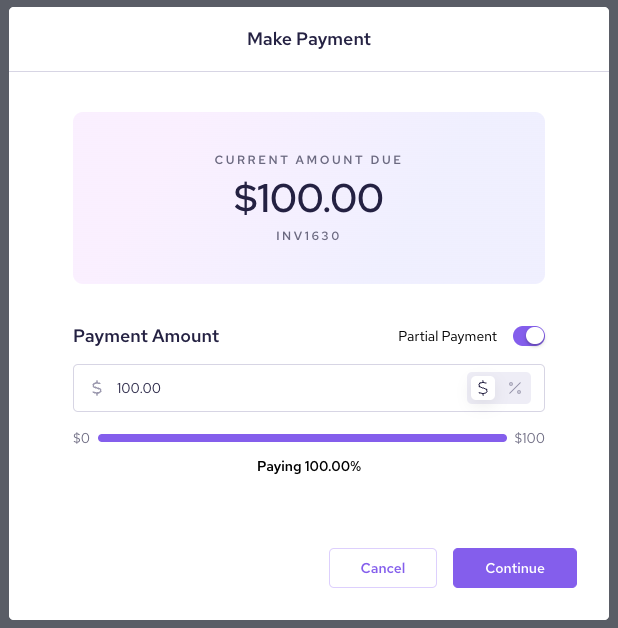
Hide Existing Payment Details
The default behaviour for the Helcim system when a payment is processed is to create a customer
and invoice
object, then tokenize and store the customers payment details within the customer
object.
When an existing customerCode
is passed to HelcimPay.js during initialization, any existing payment details for that customer are displayed in the modal by default to make the checkout process faster.
To disable this default display and require the customer to enter new payment information, you can pass a value of "1" for the hideExistingPaymentDetails
parameter in your HelcimPay.js initialize request. Existing payment details and default payment selections will remain on the customer
object, but will be hidden within the payment modal.
Set Payment Details as Default for Customer
If a customer is for the first time, their first payments of each payment method (Credit Card and ACH) will set the relevant payment details used as the default for display and processing when using that method in future.
HelcimPay.js can be used to add new credit card and ACH bank payment details for existing customers, by using the "verify" paymentType
and passing a value of "1" for the setAsDefaultPaymentMethod
parameter.
Setting new payment details as default results in:
- The entered payment details being displayed as the default in HelcimPay.js for future checkout sessions that are initialized with that customers
customerCode
. - The default payment details entered being used for other automated Helcim payment tools, such as Recurring Payments.
Processing with Fee Saver Through HelcimPay.js
Before initializing your HelcimPay.js checkout session with Helcim Fee Saver you will need to enable this service at a global level within your Helcim account to avoid errors when attempting to process payments.
Follow the instructions below and pass on your credit card processing fees through HelcimPay.js!
Confirm Invoicing is set to Credit Card and ACH
- Log in to your Helcim account.
- Go to
All Tools
and thenSettings
. - Select
ACH Bank Payment Settings
under Payments and Plans. - Ensure
Enable ACH Bank Payments
is toggled on.
- Ensure
ACH Bank Payment Tools
has invoicing set to Credit Card and ACH Payment and is toggled on.
Toggle on Helcim Fee Saver
- Go to
All Tools
and thenSettings
. - Select
Fee Saver Settings
under Payments and Plans. - Toggle the Online Fee-Saver option on.
Processing with Fee-Saver through HelcimPay.js.
Now that your Helcim account is configured to process with Fee-Saver, you will need to Initialize your HelcimPay.js checkout session with the appropriate parameters.
In addition to the required body parameters of paymentType
, amount
, and currency
, you will also need to pass the appropriate parameters of paymentMethod
as "cc-ach" and hasConvenienceFee
as "1".
With these parameters included, the HelcimPay.js payment modal will render with the appropriate convenience fee displayed for credit card processing and give customers the options to instead process through ACH payment to avoid that fee.
Select a Processing Terminal for Payment
Certain Helcim merchants may have more than one merchant account with Helcim, resulting in multiple processing terminals that are routed to unique bank accounts.
In the instance that your business with Helcim is configured in this manner, you can utilize the terminalId
parameter for HelcimPay.js initialization requests to determine with processing terminal (and subsequently which bank account) your payments are processed on and then deposited into.
To retrieve your available processing terminals and their id
values, you can use the Get card terminals endpoint in the Card Terminal API.
HelcimPay.js Initialize Response
The Helcim system will respond to a successful initialize request with a JSON object containing a checkoutToken
and secretToken
that can be used to render the HelcimPay.js checkout modal and validate any transaction that is processed.
The checkoutToken
and secretToken
returned by the HelcimPay.js initialize endpoint are only valid for 60 minutes after being returned. After this they expire and you would need to call the initialization endpoint to receive new tokens to render the HelcimPay.js modal.
The table below details the response payload received from the API upon successful execution.
{
"secretToken": "1d4b6437a8aabfe4b0ed93",
"checkoutToken": "49702e8e38d5db9226b54f"
}
Property | Type | Description |
---|---|---|
checkoutToken | String | The checkoutToken is the key to displaying the HelcimPay.js modal using the appendHelcimIframe() function. This token ensures a secure connection between the cardholderβs web browser and the Helcim Payment API endpoint. Please note, the checkout token is a unique value for each payment instance, and it expires after 60 minutes, or once the transaction is processed. Having unique and recent checkout tokens reduces the likelihood that an unauthorized payment is processed. |
secretToken | String | The secretToken is used for validation purposes after a transaction has been processed successfully. This token, along with transaction data in the response are used to create a hash. You can use this to verify that the data in the transaction response is valid and has not been tampered with. |
HelcimPay.js Initialize Endpoints
Create a HelcimPay.js checkout session using a single API call to control the features and functionality rendered in the payment modal, using the following endpoint.
Updated 3 days ago