Initiating a Purchase Transaction
Send a payment request from your existing third-party software to your registered Smart Terminal devices
Once your Smart Terminal is paired to your third-party point of sale system or software, by saving the device code in your system or making it otherwise available for transaction requests, you can initiate a Purchase Transaction that is sent to your device via the Smart Terminal API.
A Purchase Transaction sent to a Smart Terminal device can be paid by the end customer using either an Interac Debit Card or a Credit Card.
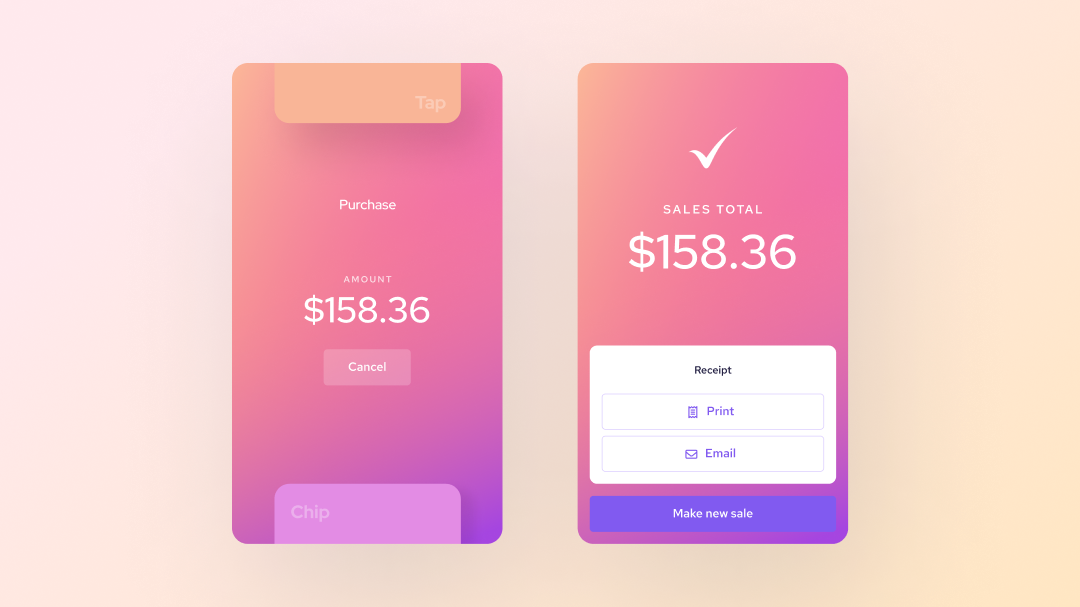
Building your Smart Terminal API Purchase Transaction Request
In order to initiate a Purchase Transaction from your existing software, a POST request should be sent to the Smart Terminal API Purchase endpoint from your systems secure back end server.
Required Parameters
Headers
api-token
: The API token for the Helcim account your devices are registered with.idempotency-key
: The unique 25 character alphanumeric key associated with this transaction.
Path Parameters
code
: Your 4 digit alphanumeric device code.
Body Parameters
currency
: The currency that you would like to process the transaction in. This should match the core currency of your business location (E.g., Canadian merchants = CAD and United States merchants = USD).transactionAmount
: The amount that you would like to process.
Optional Parameters
invoiceNumber
: TheinvoiceNumber
that you would like the transaction to be associated with. If theinvoiceNumber
exists in your Helcim account, the payment will be linked to that Invoice. If it does not exist, the Invoice object created by the transaction event will be assigned thatinvoiceNumber
. If omitted from your request, the Helcim system will create one.customerCode
: ThecustomerCode
that you would like the transaction to be associated with. If thecustomerCode
exists in your Helcim account, the payment will be linked to that Customer. If it does not exist, the Customer object created by the transaction event will be assigned thatcustomerCode
. If omitted from your request, the Helcim system will create one.
If your request is successful, the Smart Terminal will prompt the credit / debit flow for the payment on the device. The customer can then tap or use chip + PIN to complete their payment.
Example Request
To build your Purchase Transaction request in your preferred language, visit our API reference page here.
const options = {
method: 'POST',
headers: {
accept: 'application/json',
'idempotency-key': 'test-helcim-20240405-001',
'content-type': 'application/json',
'api-token': 'API_TOKEN'
},
body: JSON.stringify({currency: 'CAD', transactionAmount: 100.99})
};
fetch('https://api.helcim.com/v2/devices/FD4Y/payment/purchase', options)
.then(response => response.json())
.then(response => console.log(response))
.catch(err => console.error(err));
The Smart Terminal API does not handle duplicate Purchase transaction requests, sent to the same device, at the same time.
Because there is no queue to let your software know that a payment is already pending, you would get successful responses from subsequent requests while the device is currently processing.
Purchase Transaction Responses
202 Accepted
: Returned when a request to the Smart Terminal API was formed correctly and processed. Does not indicate that the requested action was completed.403 Unauthorized
: Returned when theapi-token
sent in the header of your request was incorrect, or does not have the valid permissions to complete the request.404 Device Not Found
: Returned when thecode
sent in the path parameters was not found by the API. This is likely the result of an incorrectcode
being sent in the request, or the device has not yet been registered with the Helcim system.409 Device Not Listening
: Returned with thecode
sent in the path parameters exists in our system, but the device is not reachable. This is likely due to the device being powered off, not logged, or being asleep.500 Internal Server Error
: Returned when the Smart Terminal API encountered an unknown error when trying to process your request.
Frequently Asked Questions
Where can I find transactions processed through the Smart Terminal in my payment list?
- Purchase Transactions processed on a Smart Terminal device through the Smart Terminal API will appear in your Payments list with the Payment Source of Helcim Card Reader and can be filtered to using the filtering tools.
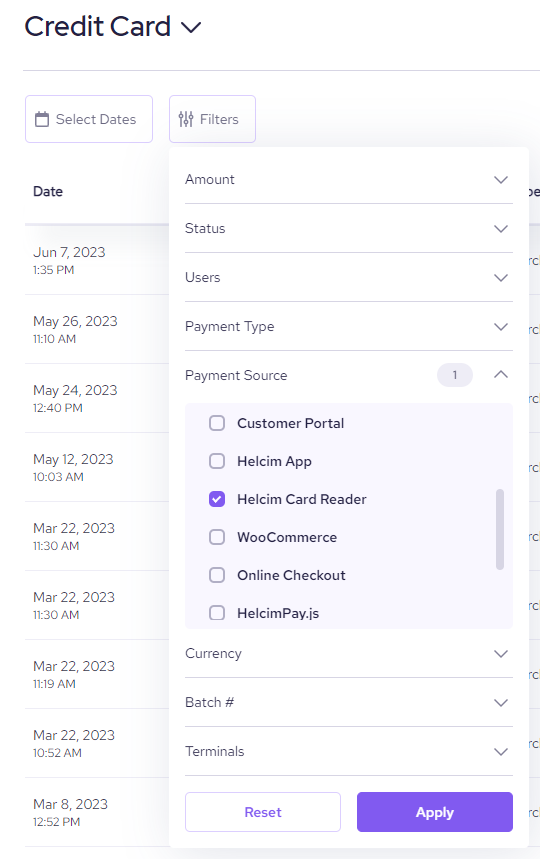
Updated 1 day ago