Webhooks
Webhooks are available for Card Transaction events for Helcim merchants who wish to be notified when a payment is processed through any Helcim payment tool. The event will trigger for both approved and declined payments, ensuring you can keep your system up to date for successful payments, or attempt to process again if a payment declined.
Helcim webhooks will send a POST request to a predetermined endpoint that you have subscribed in your webhook settings.
- The endpoint can be whatever you want, however it cannot contain the word Helcim in the URL and must have valid HTTPS in order for you to save your webhook configuration.
- The endpoint that you subscribe will be limited to one endpoint per service, and that endpoint will need to listen to all of the event types sent to it.
It's also important to disable CSRF protection for this endpoint if the framework you use enables them by default.
Another important aspect of handling webhooks is to verify the signature and timestamp when processing them. You can learn more about this in the Verifying Webhook Events section below.
Configuring webhooks
Follow the instructions below to enable and configure webhook events for your transactions.
- Log in to your Helcim account.
- Click
All Tools
. - Click
Integrations
. - Click
Webhooks
. - Toggle
Webhooks ON
.

- Enter your Deliver URL, where you would like webhooks events to be delivered.
Webhook URL requirements
The Helcim system will not accept a Deliver URL that contains the word Helcim or does not include a valid https protocol, indicating a secure URL. Ensure that your Deliver URL meets these requirements in order to save your webhook settings.
- Notify events for Transactions should be ticked.
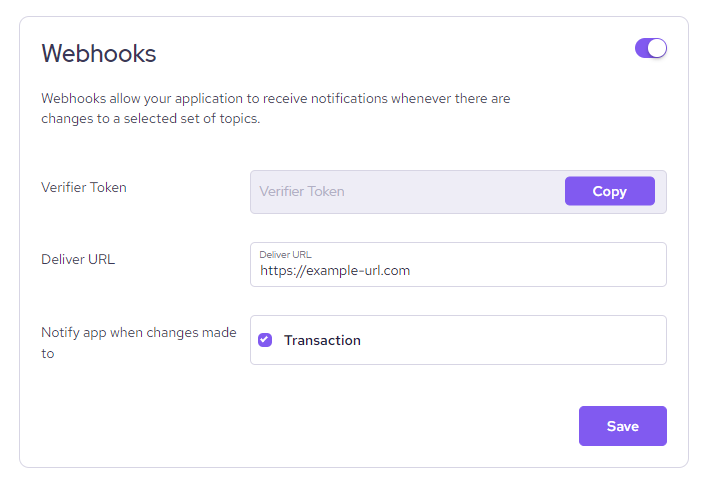
- Click
Save
.
Webhook events
A webhook event will be sent to the third-party URL that was subscribed, and will contain the id
for the relevant object, as well as the type
of event object that was sent, such as a cardTransaction
for a Smart Terminal API transactions.
Webhook events will be triggered for all events of the selected type when:
- You have subscribed to webhooks.
- An approved or declined debit or credit card payment has been processed through a Helcim payment tool, including on an API Mode enabled Smart Terminal.
// Example webhook event payload
// headers
webhook-signature:v1,CsvqmJB7JYdg74tlxbIdXe63H62QMOrMALNw51V/uYU=
webhook-timestamp:1716412291
webhook-id:msg_2gq5VYqF4DlzM66mCpaXtsEBAkp
// body
{"id":"25764674","type":"cardTransaction"}
Verifying a webhook event
Helcim uses an HMAC with SHA-256 to sign its webhooks. Webhook events are delivered with a unique webhook-signature
in the header of the request, that can be used for verification that the event being received is legitimate.
To verify the expected signature in the webhook event, you should HMAC the signedContent. This value should be the webhook-id, webhook "${webhook_id}.${webhook_timestamp}.${body}" using the base64 of your signing verifierToken
, found in your webhooks settings section of your Helcim account.
The value returned by this function should match the webhook-signature
value returned in the heading of the event.
const crypto = require('crypto');
// list of space delimited signatures and their corresponding version identifiers. The signature list is most commonly of length one. Though there could be any number of signatures.
let webhook_signature = "v1,g0hM9SsE+OTPJTGt/tmIKtSyZlE3uFJELVlNIOLJ1OE= v2,MzJsNDk4MzI0K2VvdSMjMTEjQEBAQDEyMzMzMzEyMwo="
// The content to sign is composed by concatenating the id, timestamp and payload, separated by the full-stop character (.)
signedContent = `${webhook-id}.${webhook-timestamp}.${body}`
const verifierToken = "CHANGE_ME";
// Need to base64 decode the verifierToken
const verifierTokenBytes = new Buffer(verifierToken, "base64");
// This generated signature should match one of the ones sent in the webhook-signature header.
const generated_verification_signature = crypto
.createHmac('sha256', verifierTokenBytes)
.update(signedContent)
.digest('base64');
// check that signature matches the signature sent from the header of the webhook event
if webhook_signature != generated_verification_signature) {
// reject the request
} else {
// accept the request, store data as needed
}
Confirming receipt of a webhook event
In order to confirm a webhook event was received and valid, you should respond to the event to indicate that it has been processed by returning a 2xx (status code 200-299) response to the webhook message within a reasonable time-frame.
Webhook event schedule
A failed webhook event is attempted based on the following schedule, where each period is started following the failure of the preceding attempt:
- Immediately
- 5 seconds
- 5 minutes
- 30 minutes
- 2 hours
- 5 hours
- 10 hours
- 10 hours (in addition to the previous)
For example, an event that fails three times before eventually succeeding will be delivered roughly 35 minutes and 5 seconds following the first attempt.
Retrieving transaction objects through the Card Transaction API
The webhook event sent to your subscribed URL will contain the transactionId
of the relevant Transaction object.
The webhook event sent to your subscribed URL will contain the transactionId
of the relevant Transaction object. The full transaction
object can be retrieved through the Get Card Transaction by ID endpoint.
Updated 8 days ago