HelcimPay.js Initialization
This reference outlines the steps for initializing a HelcimPay.js checkout session.
In order to integrate and render HelcimPay.js, you must first initialize your checkout session to obtain the checkoutToken for rendering the payment iFrame covered in the implementation process and the secretToken for validating the transaction response covered in the validation process.
Important Note:
When initializing HelcimPay, this request should be made from your website or applications backend server to ensure a secure connection to the Helcim API.
Initialization Request
The checkoutToken
and secretToken
returned by the HelcimPay initialize endpoint are only valid for 60 minutes after being returned. After this they expire and you would need to call the initialization endpoint to receive new tokens to render the HelcimPay modal.
The table below outlines the required headers for the request.
Property | Type | Necessity | Description |
---|---|---|---|
api-token | String | Required | The API Access token. Refer to this support article. |
The table below outlines the required payload for the request.
Property | Type | Necessity | Description |
---|---|---|---|
paymentType | String | Required | The type of transaction. verify | purchase | preauth |
amount | Number | Required | The final amount of the transaction to process. |
currency | String | Required | The currency of which the transaction will be processed. CAD | USD |
customerCode | String | Optional | An existing Helcim customer’s code you want to tie the transaction with. If this field is not present, a new customer will be created and linked to the transaction using card holder information entered during processing. |
invoiceNumber | String | Optional | An existing Helcim due invoice number you want to process. If this field is not present, a new invoice will be created and linked to the transaction. |
paymentMethod | String | Optional | The payment method that you are allowing the customer to use to pay for the amount. This could be credit card, ACH, or both. cc | ach | cc-ach |
allowPartial | Number | Optional | Passing a value of 1 will determine whether the partial payment UI will be displayed to the customer in the HelcimPay modal. |
hasConvenienceFee | Number | Optional | Passing a value of 1 will determine whether Helcim Fee Saver will be available to the customer in the HelcimPay modal, allowing for the addition of a 3% convenience fee to cover processing fees through Credit Card. |
taxAmount | Number | Optional | Passing a dollar amount for tax, to 2 decimal places, is used by Helcim to facilitate lower per transaction rates by enabling Level 2 processing. The Helcim payments system will take care of the rest. |
const payload = {
method: 'POST',
headers: {
accept: 'application/json',
'api-token': API_TOKEN,
'content-type': 'application/json'
},
body: JSON.stringify({paymentType: 'purchase', amount: 100, currency: 'CAD'})
}
axios.post('https://api.helcim.com/v2/helcim-pay/initialize', payload)
.then(response => console.log(response))
.catch(err => console.error(err));
curl --request POST \
--url https://api.helcim.com/v2/helcim-pay/initialize \
--header 'accept: application/json' \
--header 'api-token: API_TOKEN' \
--header 'content-type: application/json' \
--data '
{
"paymentMethod": "cc",
"allowPartial": 1,
"hasConvenienceFee": 1,
"paymentType": "purchase",
"amount": 100.99,
"currency": "CAD",
"customerCode": "CST1000",
"invoiceNumber": "INV1000"
}
'
Initialization Response
The HelcimPay.js Initialization endpoint will respond to a successful request with a JSON object containing a secretToken
and checkoutToken
that can be used to validate and render the HelcimPay checkout modal.
The table below details the response payload received from the API upon successful execution.
Property | Type | Description |
---|---|---|
checkoutToken | String | The checkoutToken is the key to displaying the HelcimPay.js modal using the appendHelcimIframe function. This token ensures a secure connection between the cardholder’s web browser and the Helcim Payment API endpoint. Please note, the checkout token is a unique value for each payment instance, and it expires after 60 minutes, or once the transaction is processed. Having unique and recent checkout tokens reduces the likelihood that an unauthorized payment is processed. |
secretToken | String | The secretToken is used for validation purposes after a transaction has been processed successfully. This token, along with transaction data in the response are used to create a hash. You can use this to verify that the data in the transaction response is valid and has not been tampered with. |
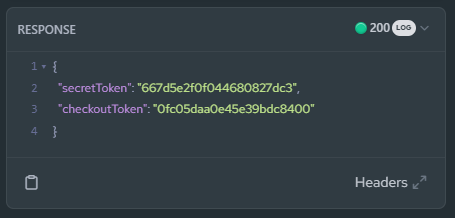
Example response from a successful initialization request
Testing Initialization:
You can test the HelcimPay initialization request and response process through our API reference documentation with the Create a HelcimPay.js Checkout Session endpoint.
Updated 4 months ago